How To Modify Controls At Runtime
You can modify the UI components and controls (especially their properties) at runtime thanks to ExeOutput's HEScript script engine.
Set and get property values¶
HEScript provides you with two functions to do so:
- SetUIProp: sets the value of the specified property of a control whose name is given by id.
- GetUIProp: gets the value of the specified property of a control whose name is given by id.
procedure SetUIProp(const id, propname, propval: String);
- id: name of the component followed by the name of the control.
- propname: name of the property.
- propval: new value to give (always a string).
function GetUIProp(const id, propname: String): String;
- id: name of the component followed by the name of the control.
- propname: name of the property.
- result: the value of the property (in string format). If the control is not found, an error may occur.
How to determine the ID of a control¶
In ExeOutput, a control's ID is defined by the name of the parent component followed immediately by the name of the control (without space between them).
For instance, for a Timer1
control that belongs to the timer1
component, its ID would be timer1Timer1
.
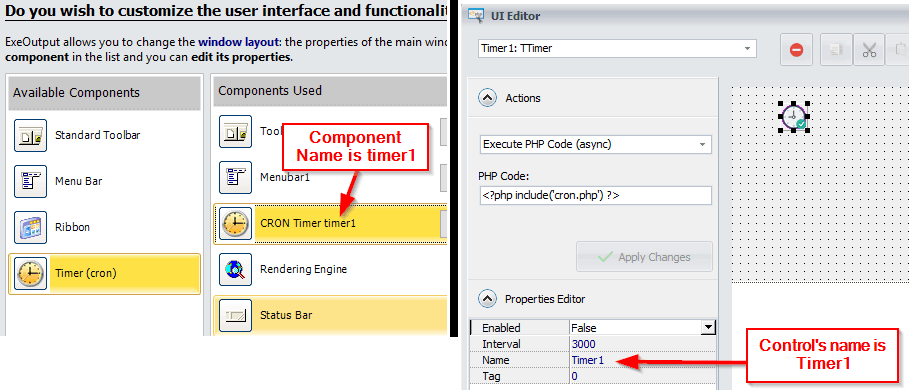
How to change a control's caption¶
The Home button's caption will be changed by the following HEScript code:
procedure Procedure1;
begin
SetUIProp("ribbon1BHome", "Caption", "TEST!!");
ShowMessage("This is Procedure 1 from UserMain script. I changed the Home button caption!");
end;
In the code above, ribbon1BHome
indicates that we should modify a property of the UI control BHome
that belongs to the ribbon1
UI component. Caption
is the name of the property and the last parameter is the value we want to set.
Note
All properties of a control listed in the Properties Editor can be modified programmatically (Caption, Visible, Enabled...).
How to show or hide a control¶
The following HEScript code will hide the control named UserLabel
:
procedure Procedure2;
begin
SetUIProp("ribbon1UserLabel", "Visible", "False");
end;
The same for making it visible:
procedure Procedure2;
begin
SetUIProp("ribbon1UserLabel", "Visible", "True");
end;
To execute these HEScript procedures, please refer to the corresponding topic: how to call HEScript functions from your PHP, HTML code (links) and even from JavaScript.